Connect to Azure:
How to connect to Azure via Powershell (first time)
Requirements: * PowerShell 5.1 * .NET Framework 4.7.2 or later. * Azure Powershell Module Check Powershell Version:
# PSVersioncheck
$PSVersionTable.PSVersion Check .NET Framework Version:
# Check for .NET Framework 4.7.2 Get-ChildItem ’HKLM:\SOFTWARE\Microsoft\NET Framework Setup\NDP\v4\Full…
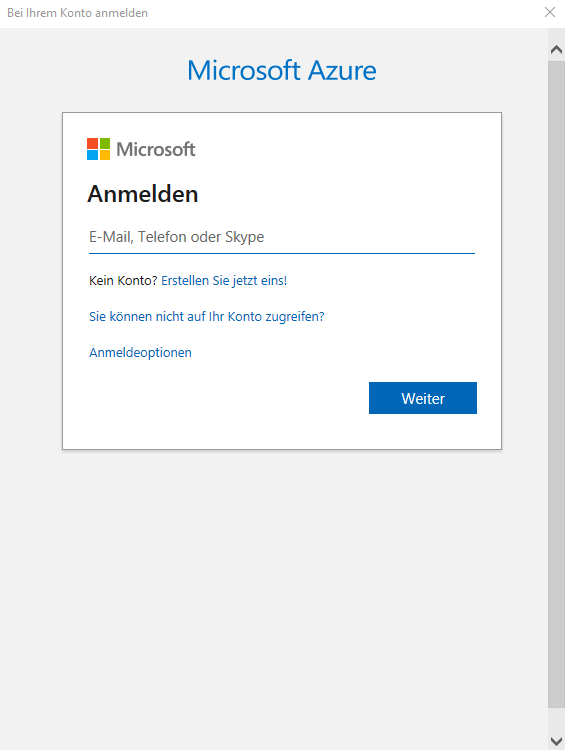
Query and choose a subscription
$Subscription = Get-AzSubscription | Out-GridView -PassThru
Select-AzSubscription $Subscription
Query and choose a resource group
$ResourceGroup = Get-AzResourceGroup | Out-GridView -PassThru
Define locktype
$lock = 'ReadOnly','CanNotDelete'
# Choose one
Define lockname
$lockSite = Read-Host -Prompt 'Please define a locksite name'
Set lock
New-AzResourceLock -LockLevel $lock -LockName $lockSite -ResourceGroupName $ResourceGroup.ResourceGroupName -Force
Get lock
$ActiveLock = Get-AzResourceLock -ResourceGroupName $ResourceGroup.ResourceGroupName | Out-GridView -PassThru
Remove lock
Remove-AzResourceLock -LockId $ActiveLock.LockId
Complete Script:
(with a little bit of automation)
############################################################################
# #
# Created by #
# #
# Powershell Skript - Create or Remove a Ressourcegrouplock #
# 30.12.2019 #
# Thomas Bründl #
# #
############################################################################
#Prequistes
# -------------------------------------------------------------------------------
# To connect to Azure:
#
# - Powershell AZ-Module
# - PSVersion 5.1
# - .Net - Framework 4.7.2 or later
# -------------------------------------------------------------------------------
# Functions
# -------------------------------------------------------------------------------
function Show-Menu
{
param (
[string]$Title = '<Value>'
)
Clear-Host
Write-Host "================ $Title ================"
Write-Host ""
Write-Host "1: CanNotDelete - Press '1' for this option." -ForegroundColor Yellow
Write-Host "2: ReadOnly - Press '2' for this option." -ForegroundColor Yellow
Write-Host "3: Remove Lock - Press '3' for this option." -ForegroundColor Yellow
Write-Host "Q: Press 'Q' to quit." -ForegroundColor Yellow
Write-Host ""
Write-Host "========================================"
}
# Source: https://4sysops.com/archives/how-to-build-an-interactive-menu-with-powershell/
# Vars
# -------------------------------------------------------------------------------
# Script
# -------------------------------------------------------------------------------
$Debug = [System.Windows.Forms.MessageBox]::Show("Do you want to activate the verbose mode?","Verbose Mode",4,[System.Windows.Forms.MessageBoxIcon]::Question)
If ($Debug -eq "Yes")
{
$VerbosePreference = 'Continue'
Write-Verbose "Verbose Mode activated!"
}
# Source: https://blog.stefanrehwald.de/2013/03/06/powershell-05-messagebox/
# Connect zu Azure
Connect-AzAccount
# Query and choose a Subscription
$Subscription = Get-AzSubscription | Out-GridView -PassThru
Write-Verbose $Subscription
Select-AzSubscription $Subscription
# Query and choose a ResourceGroup
$ResourceGroup = Get-AzResourceGroup | Out-GridView -PassThru
Write-Verbose $ResourceGroup
Show-Menu –Title 'Create or remove lock'
$selection = Read-Host 'Please make a selection'
switch ($selection)
{
'1' {
Write-Host 'You chose option #1 - CanNotDelete - lock is going to be created.' -ForegroundColor Yellow
$lock = 'CanNotDelete'
} '2' {
Write-Host 'You chose option #2 - ReadOnly - lock is going to be created.' -ForegroundColor Yellow
$lock = 'ReadOnly'
} '3' {
Write-Host 'You chose option #3 - a lock is going to be removed.' -ForegroundColor Yellow
$lock = 'NoLock'
} 'q' {
exit
}
}
Write-Verbose $lock
if ($selection -like 1 -or $selection -like 2){
$lockSite = Read-Host -Prompt 'Please define a locksite name'
Write-Verbose $lockSite
New-AzResourceLock -LockLevel $lock -LockName $lockSite -ResourceGroupName $ResourceGroup.ResourceGroupName -Force
}
if ($selection -like 3){
$ActiveLock = Get-AzResourceLock -ResourceGroupName $ResourceGroup.ResourceGroupName | Out-GridView -PassThru
Remove-AzResourceLock -LockId $ActiveLock.LockId
}
# Source: https://docs.microsoft.com/en-us/azure/azure-resource-manager/management/lock-resources
Sources:
Lock resources to prevent changes - Azure Resource Manager
Prevent users from updating or deleting critical Azure resources by applying a lock for all users and roles.
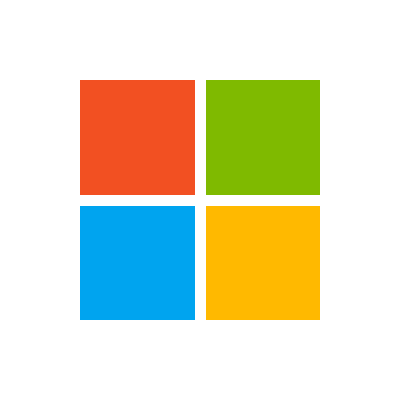
PowerShell – 05 – MessageBox
How to build an interactive menu with PowerShell
If you’re building a script that’s capable of doing various tasks simultaneously, a great way of providing input for that script is to use an interactive menu in the console.
