In this article, you will learn how to persistently store parameters for your scripts.
Prerequisites
- PSv3 (PowerShell 3.0) and up
- IDE (integrated development environment) - Power Shell ISE / Visual Studio Code
Introduction:
With almost every project that is based on PowerShell, I ask myself how and where I collect the necessary parameters.
Normally they are fixed defined or collected interactively during the execution of the script.
What if, the person executing the script is not that experienced with computers and is simply overwhelmed with these questions?.
What if I want to pick up exactly those people and provide them a self-service tool in order to avoid overwhelming them with too many questions and still give them the opportunity to help themselves?
My proposed solution here is to use configuration files.
In a historical overview, every experienced sysadmin will be familiar with the 3 common formats:
- INI (INItialization file)
- XML (Extensible Markup Language)
- JSON (JavaScript Object Notation)
State of the art is certainly the use of JSON.
This is the topic I would like to address in this blog post.
Let's get some hands-on experience:
First we start our favourite IDE (integrated development environment), in my case Visual Studio Code. If you don't want to install anything, Power Shell ISE, which is pre-installed with Windows, can be used as well.
First we define an example JSON configuration. For this, I create an array and fill it with the necessary information.
# Example JSON - Array
$data=@"
{
"computer1": {
"name": "Comp1",
"temppath": "C:\\Temp",
"components": {
"graphic": "AMD Radeon",
"discs": [
"C:\\ 100GB",
"D:\\ 200GB"
],
"lan": "100 Mbit"
},
"support": {
"email": "t.bruendl@it-infrastructure.solutions"
}
}
}
"@
Subsequently, we import this information and create a PS object from it.
# Converting JSON-Array to PS-Object
$json=$data | ConvertFrom-Json
Now we want to retrieve the attributes of the newly created PS object.
# Testing PS-Object attributes
$json.computer1.name
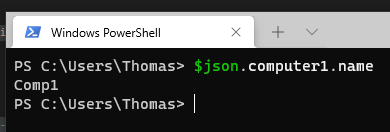
Of course, you can also edit these attributes....
# Adjusting the attributes
$json.computer1.name = "Comp2"
$json.computer1.name
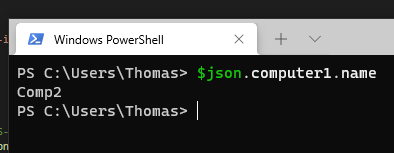
Finally, of course, you want to save these changes persistently.
# Export to file
$json | ConvertTo-Json -depth 100 | Out-File "C:\Users\Thomas\Desktop\Test.json"

And how do you retrieve the parameters stored in this way?
# Import from file to a new PS-Object
$test=Get-Content -Path "C:\Users\Thomas\Desktop\Test.json" -Raw | ConvertFrom-Json

Last check if everything has worked and the values are available.
# Testing PS-Object attributes
$test.computer1.name
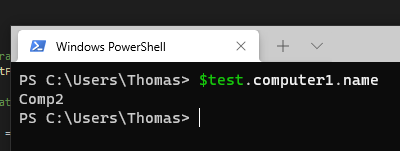
Conclusio:
You should now know how to save parameters persistent.
At a next step I plan to create a module on this basis.
I think of commands like:
Get-Configuration
Set-Configuration
Update-ConfigurationParameter
And so on....
So stay tuned for Part 2.....
Sources:
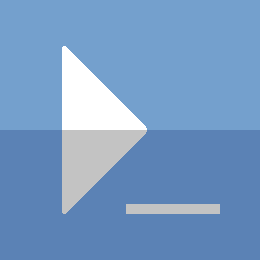
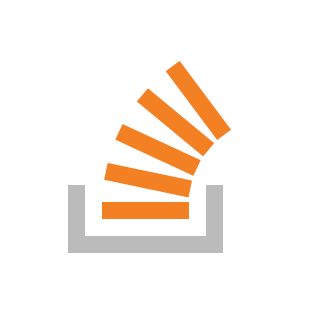
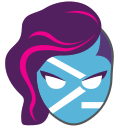